I thought I'd make this project to show how much you can do with vanilla javascript without any frameworks or templating engines (eg: react, vuejs, ember, jsx)
All code for this project is on github
Getting an API Key #
In order to make any requests we will need to signup to unsplash as a developer & request an API key.
Basic Design #
The first thing to do is get a basic idea of what we want to make.
Things we will need:
- Title
- Search Form
- Results Section
<body>
<h1>Unsplash Search</h1>
<form>
<label for="searchTerm">Image Search</label>
<input class="searchBox" type="text" id="searchTerm" name="searchTerm" />
<button type="submit">Search</button>
</form>
<section class="images" />
<div class="createdby">
<p>
created by <a href="https://github.com/equk">equk</a>
</p>
</div>
</body>
created basic html sructure with some css styles a279fd7
Adding Javascript #
Start with some basic consts for using the unsplash API & the selector for the output div.
public/js/app.js
const API_CLIENTID = "-insert-your-api-key-";
const form = document.querySelector("form");
const input = document.querySelector("input");
const imageSection = document.querySelector(".images");
const API_URL = `https://api.unsplash.com/search/photos?page=1&per_page=20&client_id=${API_CLIENTID}`;
added js file with some basic const variables 40692d7
First API Request #
Now we want to setup a request to pull in some json using the API.
We will use the fetch()
API as it will let us use promises utilizing async requests.
At first we just log this to the console to test the request returns something we can parse.
- const API_CLIENTID = '-insert-your-api-key-'
+ const API_CLIENTID = ''
const form = document.querySelector('form');
const input = document.querySelector('input');
const imageSection = document.querySelector('.images');
const API_URL = `https://api.unsplash.com/search/photos?page=1&per_page=20&client_id=${API_CLIENTID}`
+
+ form.addEventListener('submit', formSubmitted);
+
+ function formSubmitted(event) {
+ event.preventDefault();
+ let searchTerm = input.value;
+
+ search(searchTerm)
+ }
+
+ function search(searchTerm) {
+ let url = `${API_URL}&query=${searchTerm}`;
+ return fetch(url)
+ .then(response => response.json())
+ .then(result => {
+ console.log(result.results);
+ });
+ }
setup a fetch request to pull in json & log to console 3b4106e
So far we have javascript which sends a request using the fetch()
API which creates a promise
that resolves a response
& writes it to the console
.
Parsing API Request #
Next we want to parse the API request to something usable.
At first we just want to output some images to the output div.
In order to do this with vanilla javascript we will use DOM Manipulation (something most old javascript developers will have used before).
event.preventDefault();
let searchTerm = input.value;
+ searchStart();
search(searchTerm)
+ .then(displayImages)
+ }
+
+ function searchStart() {
+ imageSection.innerHTML = '';
}
function search(searchTerm) {
let url = `${API_URL}&query=${searchTerm}`;
return fetch(url)
.then(response => response.json())
.then(result => {
- console.log(result.results);
+ return result.results;
});
}
+
+ function displayImages(images) {
+ images.forEach(image => {
+ let imageElement = document.createElement('img');
+ imageElement.src = image.urls.regular;
+ imageSection.appendChild(imageElement);
+ });
+ }
added function to display images using DOM manipulation cdaf141
We now have some images appearing in the output div but they are all over the place so lets set some CSS for each item turning them into a grid of square images.
public/css/app.css
+ img {
+ object-fit: cover;
+ width:350px;
+ height:350px;
+ padding: 0 4px;
+ }
styled images to be small boxes 350px with grid padding 2813e4d
Unsplash API Terms #
Looking into the Unsplash API Terms there are some rules regarding attribution.
Unsplash API Terms (Attribution)
Each time you or your Developer App displays a Photo, your Developer App must attribute Unsplash, the Unsplash photographer, and contain a link back to the photographer’s Unsplash profile.
Adding Attribution Information #
Lets parse more data from the API response and add in more fields (unsplash link, user, profile link).
public/js/app.js
function displayImages(images) {
images.forEach(image => {
let imageContainer = document.createElement("div");
imageContainer.className = "ImageResult";
imageContainer.innerHTML = `<img src="${image.urls.regular}">
<a href="${image.links.html}" target="_blank" class="view_link">View on Unsplash</a>
<a href="${user.links.html}" target="_blank" class="user_link">Photo by: ${image.user.name}</a>`;
imageSection.appendChild(imageContainer);
});
}
re-structured displayImages using innerHTML bbda79b
Final Design #
We now have a project that is able to send requests to a json api using a valid id utilizing promises (async) & return a gallery of results using DOM manipulation.
This is all done in vanilla javascript without the need of any frameworks (jquery, react etc).
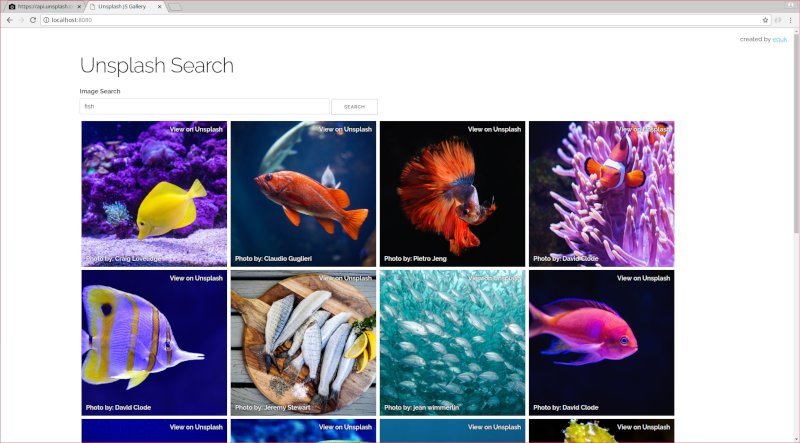
View the project on github.
References: fetch() / vanillajs / promises / DOM / Unsplash API
Webmentions
No Comments Yet